Non utilizzare un codice di blocco con ECB per la crittografia simmetrica
(Si applica a AES, 3DES, ...)
Ecco un post e molto simile < a href="http://support.microsoft.com/kb/317535"> Articolo di Microsoft KB su come la modalità ECB produce codice non crittografato.
Vedi anche questo post simile da Rook
Messaggio di testo normale:
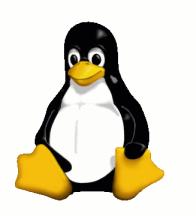
LostessomessaggiocrittografatoconlamodalitàECB(nonimportaqualecifrausi):
Lo stesso messaggio ESATTO usando la modalità CBC (di nuovo, non importa quale cifra usi):

Laviasbagliata
publicstaticstringEncrypt(stringtoEncrypt,stringkey,booluseHashing){byte[]keyArray=UTF8Encoding.UTF8.GetBytes(key);byte[]toEncryptArray=UTF8Encoding.UTF8.GetBytes(toEncrypt);if(useHashing)keyArray=newMD5CryptoServiceProvider().ComputeHash(keyArray);vartdes=newTripleDESCryptoServiceProvider(){Key=keyArray,Mode=CipherMode.ECB,Padding=PaddingMode.PKCS7};ICryptoTransformcTransform=tdes.CreateEncryptor();byte[]resultArray=cTransform.TransformFinalBlock(toEncryptArray,0,toEncryptArray.Length);returnConvert.ToBase64String(resultArray,0,resultArray.Length);}
L'erroreènellarigaseguente
{Key=keyArray,Modalità=CipherMode.ECB,Padding=PaddingMode.PKCS7};
Laviagiusta
IbraviragazzidiMicrosoftmihannoinviatoilseguentecodicepercorreggerel'articolodellaKnowledgeBasecollegatoinprecedenza.Questoèriferitonelcaso#111021973179005
QuestocodicediesempioutilizzaAESpercrittografareidatielachiaveperlacrittografiaAESèilcodicehashgeneratodaSHA256.AESèl'algoritmoAdvancedEncryptionStandard(AES).L'algoritmoAESèbasatosupermutazioniesostituzioni.Lepermutazionisonoriarrangiamentideidatielesostituzionisostituisconoun'unitàdidaticonun'altra.AESeseguepermutazioniesostituzioniusandodiversetecnichediverse.PerulterioridettaglisuAES,consultarel'articolo"Proteggi i tuoi dati con il nuovo standard avanzato di crittografia" su MSDN Magazine all'indirizzo link .
SHA è Secure Hash Algorithm. SHA-2 (SHA-224, SHA-256, SHA-384, SHA-512) è ora raccomandato. Per informazioni più dettagliate sui valori hash in .NET Framework, fai riferimento al link .
Il valore predefinito della modalità per l'operazione dell'algoritmo simmetrico per AesCryptoServiceProvider
è CBC. CBC è la modalità Cipher Block Chaining. Introduce feedback. Prima che ogni blocco di testo normale sia crittografato, viene combinato con il testo cifrato del blocco precedente mediante un'operazione OR esclusiva bit a bit. Ciò garantisce che, anche se il testo semplice contiene molti blocchi identici, verranno crittografati ciascuno in un diverso blocco di testo cifrato. Il vettore di inizializzazione è combinato con il primo blocco di testo normale mediante un'operazione OR esclusiva per bit prima che il blocco venga crittografato. Se un singolo bit del blocco di testo cifrato viene manomesso, anche il corrispondente blocco di testo in chiaro viene alterato. Inoltre, un pezzettino nel blocco successivo, nella stessa posizione del morso storpiato, verrà distrutto. Per informazioni più dettagliate su CipherMode
, fai riferimento a link .
Ecco il codice di esempio.
// This function is used for encrypting the data with key and iv.
byte[] Encrypt(byte[] data, byte[] key, byte[] iv)
{
// Create an AESCryptoProvider.
using (var aesCryptoProvider = new AesCryptoServiceProvider())
{
// Initialize the AESCryptoProvider with key and iv.
aesCryptoProvider.KeySize = key.Length * 8;
aesCryptoProvider.IV = iv;
aesCryptoProvider.Key = key;
// Create encryptor from the AESCryptoProvider.
using (ICryptoTransform encryptor = aesCryptoProvider.CreateEncryptor())
{
// Create memory stream to store the encrypted data.
using (MemoryStream stream = new MemoryStream())
{
// Create a CryptoStream to encrypt the data.
using (CryptoStream cryptoStream = new CryptoStream(stream, encryptor, CryptoStreamMode.Write))
// Encrypt the data.
cryptoStream.Write(data, 0, data.Length);
// return the encrypted data.
return stream.ToArray();
}
}
}
}
// This function is used for decrypting the data with key and iv.
byte[] Decrypt(byte[] data, byte[] key, byte[] iv)
{
// Create an AESCryptoServiceProvider.
using (var aesCryptoProvider = new AesCryptoServiceProvider())
{
// Initialize the AESCryptoServiceProvier with key and iv.
aesCryptoProvider.KeySize = key.Length * 8;
aesCryptoProvider.IV = iv;
aesCryptoProvider.Key = key;
// Create decryptor from the AESCryptoServiceProvider.
using (ICryptoTransform decryptor = aesCryptoProvider.CreateDecryptor())
{
// Create a memory stream including the encrypted data.
using (MemoryStream stream = new MemoryStream(data))
{
// Create a CryptoStream to decrypt the encrypted data.
using (CryptoStream cryptoStream = new CryptoStream(stream, decryptor, CryptoStreamMode.Read))
{
// Create a byte buffer array.
byte[] readData = new byte[1024];
int readDataCount = 0;
// Create a memory stream to store the decrypted data.
using (MemoryStream resultStream = new MemoryStream())
{
do
{
// Decrypt the data and write the data into readData buffer array.
readDataCount = cryptoStream.Read(readData, 0, readData.Length);
// Write the decrypted data to resultStream.
resultStream.Write(readData, 0, readDataCount);
}
// Check whether there is any more encrypted data in stream.
while (readDataCount > 0);
// Return the decrypted data.
return resultStream.ToArray();
}
}
}
}
}
}
// This function is used for generating a valid key binary with UTF8 encoding and SHA256 hash algorithm.
byte[] GetKey(string key)
{
// Create SHA256 hash algorithm class.
using (SHA256Managed sha256 = new SHA256Managed())
// Decode the string key to binary and compute the hash binary of the key.
return sha256.ComputeHash(Encoding.UTF8.GetBytes(key));
}
Per maggiori dettagli sulle classi nel codice di esempio, fai riferimento ai seguenti link:
· Classe AesCryptoServiceProvider
· SHA256Gestione gestita
· Classe CryptoStream
Inoltre, ci sono diversi articoli che possono aiutare a comprendere meglio la crittografia in .NET Framework, fare riferimento ai collegamenti seguenti:
· Servizi di crittografia
· Modello di crittografia di .NET Framework
· Una semplice guida alla crittografia
· Crittografia senza segreti